Beginning Programming in Python
Fall 2019

Agenda
- Files
- Writing Files
- Reading files
- With keyword
- For loops on file handles
- Binary files
- Large worked example (Fasta file processing)
- Directories
- Web file retrieval
source of input
- User Input
- Slow if needed to enter a lot of data
- Error-prone: the user may enter wrong values
- What if we want to run again?
- What if we want an input based on inputs of previous executions.
- Files
- Enter data once into a file, save it, and reuse it.
- Good for large amounts of data
- Programs can use files to communicate
- Need to be able to read from and write to files
2
Advanced issues found▲
1
files
- In Python, a file operation takes place in the following order:
- Open the file
- Read or write (perform operation)
- Close the file
- Files can be opened for different purposes
- Reading, writing, updating
- Open files must be closed
- To release the resources
- To flush the output
2
Advanced issues found▲
1
opening a file
- To access a file, it must first be opened
- The "open" function is used to open a file
- <variable> = open(<path>, <mode>)
- <variable> is of type file (file is a data type!)
- <path> is the path and name of the file
- <mode> is the opening mode (read, write, update)
5
Advanced issues found▲
2
Advanced issues found▲
1
my_file = open("test.txt", "w")
opening modes
Mode | Description |
---|---|
'r' | Opens a file for reading (default) |
'w' | Opens a file for writing. Creates a new file if it does not exist |
'a' | Open for appending at the end of the file. Creates a new file if it does not exist. |
'b' | Opens in binary mode |
'+' | Opens a file for updating (reading & writing) |
closing a file
-
When we are done with operations to the file, we need to properly close the file
-
The "close" method is used to close the file
-
It can be automatically done using "with" statement
2
Advanced issues found▲
f = open('output.txt', 'w')
# some operations here
f.close()
with open("data.txt", "a+") as f:
# some operations here
# now the file has been closed
writing to a file
Reading from a file
0
Advanced issues found▲
- When a file is opened for reading, an input pointer is positioned at the beginning of the file
- After reading, the pointer is moved after the last read character
- Reading in the text mode returns data in string
- When the input pointer reaches the end of the file, any further read attempts results in an empty string
reading a file
"with" keyword
5 minutes break!
for loop on a file
Binary files
0
Advanced issues found▲
- Python can process binary files, which are just files with arbitrary bits in them
- We can always treat a text file as a binary file, it's just that we ignore formatting
with open("test.txt", "rb") as f: # Here we treat test.txt as a binary file - any file
# can be considered just a collection of bits
with open("test2.txt", "wb") as g: # Note the nested with statements
while True:
buf = f.read(1024) # The argument to read tells it to read a set number of
# bytes - each byte is an 8 bit (8 0's or 1's) words into the "buf" object,
# which is a string
if len(buf) == 0: # We're at the end
break
g.write(buf)
directory Example
Downloading from web
Example
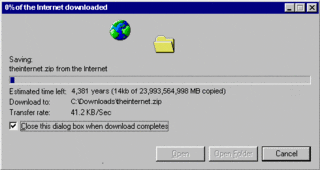
downloading data
Fasta file reading
Example

Fasta file example
Lecture 11 challenge
Questions?

CSE 20 - Lecture 11
By Narges Norouzi
CSE 20 - Lecture 11
- 1,563