Lecture 14

Fall 2018
Narges Norouzi
Review

abstract methods
- Abstract method: no implementation
-
A class containing abstract methods:
- an abstract class
- You cannot instantiate any object from an abstract classes. Why?
- If a subclass don't implement all abstract methods of its superclass, it will be abstract too
example


Interface
-
Sometimes we have an abstract class, with no concrete method
-
Interface:
-
Pure abstract class
-
No concrete method
-

Interface
Multiple inheritance
Multiple inheritance in java
- A class can inherit from one and only one class
- A class may implement zero or more interfaces
- An interface can inherit from zero or more interfaces

What about name collision?
The return types are incompatible for the inherited methods B.f(), A.f()

What about name collision?
Return types are the same and in fact we recognize only one abstract method in TestClass

name collision between interface and superclass?
why is this an error?

name collision between interface and superclass?
In name collision between parent and interface, the method in parent should be public

name collision between interface and superclass?
Why TestClass is not abstract anymore?

Interface extension
-
Interfaces may inherit other interfaces
-
Code reuse in Is-a relationship

Interface properties
-
No member variable
-
Variables: implicitly final and static
-
Usually interfaces do not declare any variables
-
- No constructor, why?

Example
"Super" keyword
Super
-
Access to parent members
-
The super reference can be used to refer to the parent class
-
super.f() invokes f() from parent class
-
Why we need it?
-
When the method is overridden in subclass
-
super is also used to invoke the parent's constructor
-
Application of super

variables in inheritance

super()
Initialization
-
Constructors are not inherited
-
even though they have public visibility
-
- We often want to use the parent's constructor to set up the "parent's part" of the object

construction
-
A child’s constructor is responsible for calling the parent’s constructor and it is done using super keyword
-
The first statement of a child’s constructor should be the super reference to call the parent constructor
-
Otherwise, default constructor is implicitly invoked
-
What if default constructor does not exist?
-
A syntax error
-
You should explicitly call an appropriate parent constructor
-
-
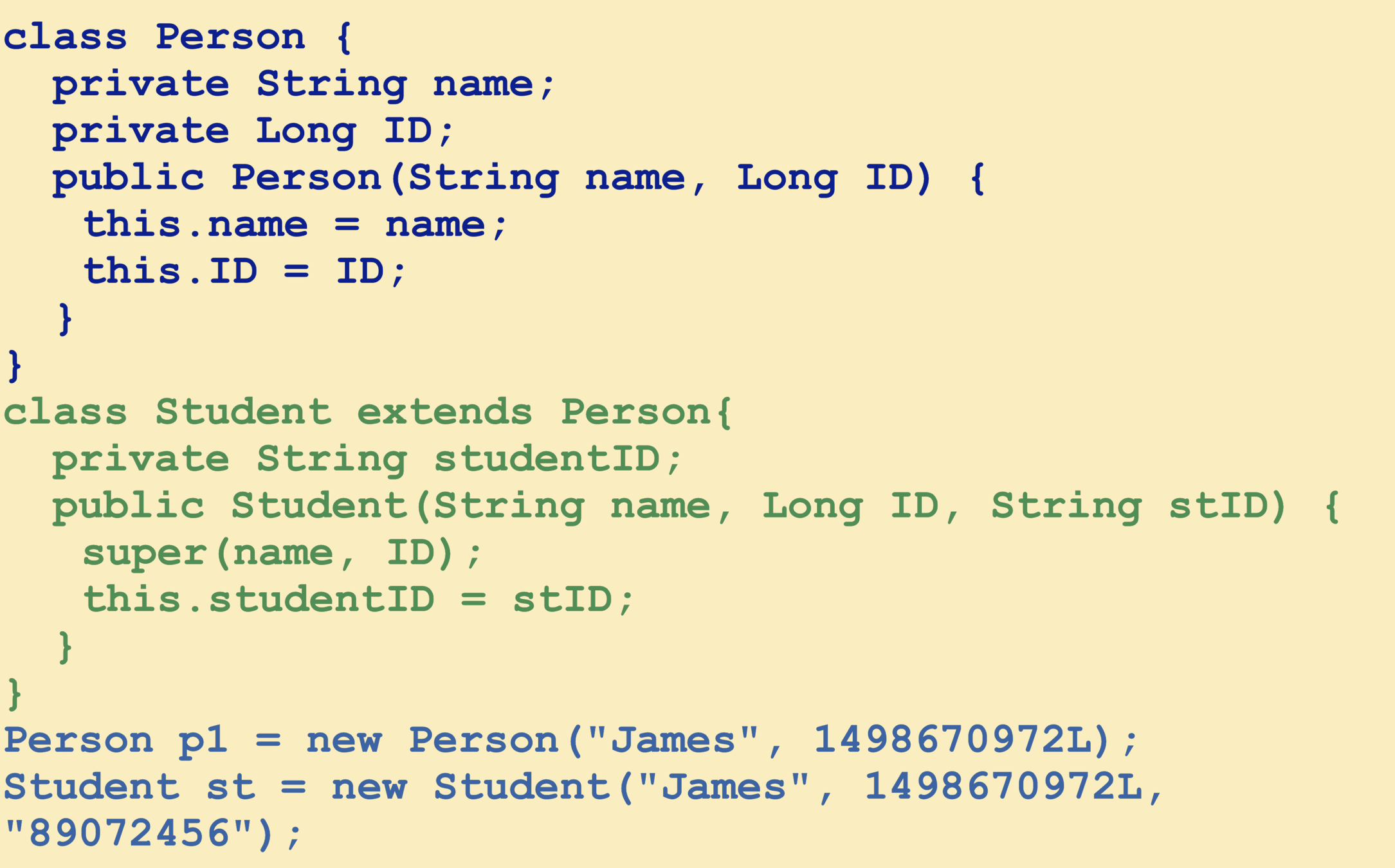
A little class activity (!)
Example 1
public class Circle extends Shape{
public static void main(String[] args){
new Shape();
}
}
class Shape{
Shape(){
System.out.println("A Shape is created!");
}
}
Example 2
public class Circle extends Shape{
public static void main(String[] args){
new Circle();
}
}
class Shape{
Shape(){
System.out.println("A Shape is created!");
}
}
Example 3
public class Circle extends Shape{
public static void main(String[] args){
new Shape();
}
Circle(){
System.out.println("A Circle is created!");
}
}
class Shape{
Shape(){
System.out.println("A Shape is created!");
}
}
Example 4
public class Circle extends Shape{
public static void main(String[] args){
new Circle();
}
Circle(){
System.out.println("A Circle is created!");
}
}
class Shape{
Shape(){
System.out.println("A Shape is created!");
}
}
Example 5
class Circle extends Shape{
Circle(){
System.out.println("A Circle is created!");
}
}
public class Shape{
Shape(){
System.out.println("A Shape is created!");
}
public static void main(String[] args){
new Circle();
}
}
Example 6
class Circle extends Shape{
Circle(){
super();
if(shapeIsCalled)
System.out.println("A Circle is created!");
}
}
public class Shape{
boolean shapeIsCalled;
Shape(){
shapeIsCalled = true;
System.out.println("A Shape is created!");
}
public static void main(String[] args){
new Circle();
}
}
Example 7
class Circle extends Shape{
boolean circleIsCalled;
Circle(){
super();
circleISCalled = true;
if(shapeIsCalled)
System.out.println("A Circle is created!");
}
}
public class Shape{
Shape(){
if(circleIsCalled)
System.out.println("A Shape is created!");
}
public static void main(String[] args){
new Circle();
}
}
Example 8
class Circle{
Circle(){
this(1);
System.out.println("A Circle is created by the 1st constructor!");
}
Circle(int x){
System.out.println("A Circle is created by the 2nd constructor!");
}
public static void main(String[] args){
Circle c = new Circle();
}
}
Example 9
class Circle extends Shape{
Circle(){
if(shapeIsCalled)
System.out.println("A Circle is created!");
}
}
public class Shape{
boolean shapeIsCalled;
Shape(){
shapeIsCalled = true;
System.out.println("A Shape is created!");
}
public static void main(String[] args){
new Circle();
}
}
Example 10
class Circle extends Shape{
Circle(){
super(1.1);
if(shapeIsCalled)
System.out.println("A Circle is created!");
}
}
public class Shape{
boolean shapeIsCalled;
Shape(double d){
shapeIsCalled = true;
System.out.println("A Shape is created! r = " + d);
}
public static void main(String[] args){
new Circle();
}
}
Example 11
class Circle extends Shape{
Circle(){
this(1.1);
System.out.println("A Circle is created by the 1st constructor!");
}
Circle(double x){
System.out.println("A Circle is created by the 2nd constructor!");
}
public static void main(String[] args){
Circle c = new Circle();
}
}
class Shape{
Shape(){
System.out.println("A Shape is created!");
}
}
Example 12
class Circle extends Shape{
Circle(){
this(1.1);
System.out.println("A Circle is created by the 1st constructor!");
}
Circle(double x){
System.out.println("A Circle is created by the 2nd constructor!");
}
public static void main(String[] args){
Circle c = new Circle();
}
}
class Shape{
Shape(double d){
System.out.println("A Shape is created!");
}
}
Example 13
class Circle extends Shape{
Circle(){
super(1.1);
this(1.1);
System.out.println("A Circle is created by the 1st constructor!");
}
Circle(double x){
System.out.println("A Circle is created by the 2nd constructor!");
}
public static void main(String[] args){
Circle c = new Circle();
}
}
class Shape{
Shape(double d){
System.out.println("A Shape is created! r = " + d);
}
}
Example 14
class Circle extends Shape{
Circle(){
this(1.1);
System.out.println("A Circle is created by the 1st constructor!");
}
Circle(double x){
super(x);
System.out.println("A Circle is created by the 2nd constructor!");
}
public static void main(String[] args){
Circle c = new Circle();
}
}
class Shape{
Shape(double d){
System.out.println("A Shape is created " + d);
}
}
Example 15
class Circle extends Shape{
Circle(double x){
super(x);
System.out.println("A Circle is created.");
}
void callMe(){
System.out.println("Circle's call me.");
}
public static void main(String[] args){
Circle c = new Circle(2.2);
}
}
class Shape{
double r;
Shape(double d){
this.r = d;
callMe();
System.out.println("A Shape is created " + d);
}
void callMe(){
System.out.println("Shape's call me.");
}
}
Example 16
class Circle extends Shape{
Circle(double x){
super(x);
System.out.println("A Circle is created.");
}
void callMe(){
System.out.println("Circle's call me.");
}
public static void main(String[] args){
Shape s = new Shape(2.2);
}
}
class Shape{
double r;
Shape(double d){
this.r = d;
callMe();
System.out.println("A Shape is created " + d);
}
void callMe(){
System.out.println("Shape's call me.");
}
}
Example 17
class Circle extends Shape{
double r = 3.3;
Circle(double x){
super(x);
}
void callMe(){
System.out.println("Circle's call me.");
}
public static void main(String[] args){
Shape s = new Shape(2.2);
}
}
class Shape{
double r = 2.2;
Shape(double d){
System.out.println("r is " + this.r);
this.r = d;
System.out.println("r is " + this.r);
callMe();
}
void callMe(){
System.out.println("Shape's call me.");
}
}
Review of assignment
Zybooks chapters we covered
-
Section 10.3: super keyword
Copy of CMPS12A - Lecture 14
By Narges Norouzi
Copy of CMPS12A - Lecture 14
- 223